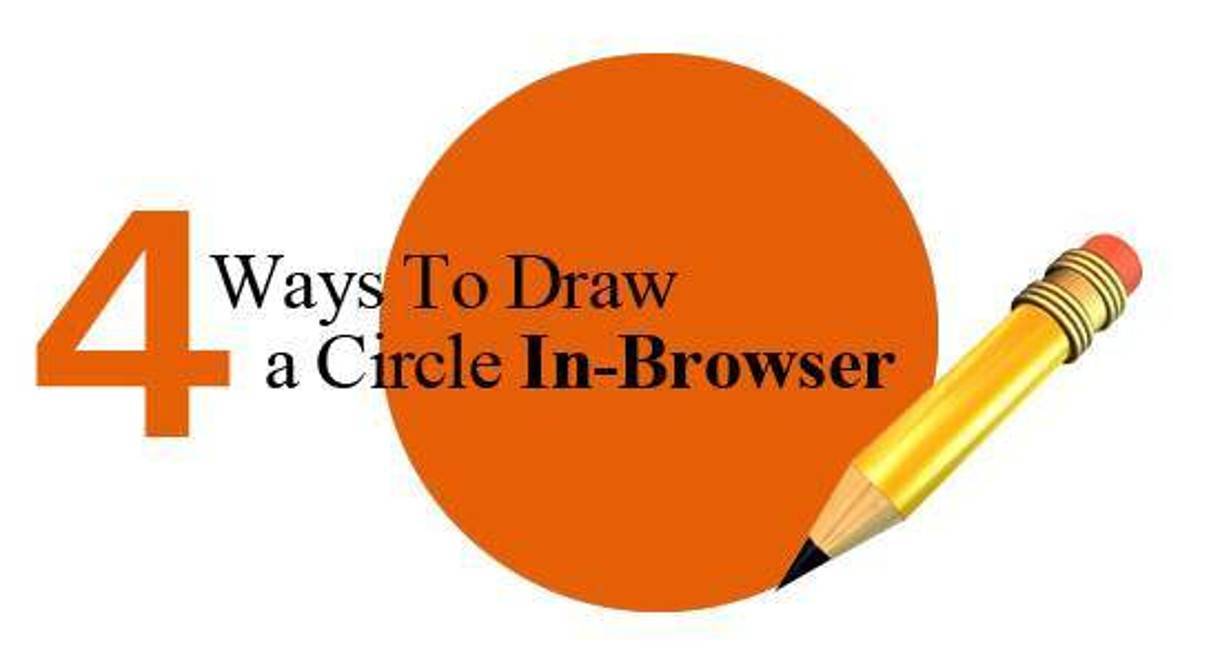
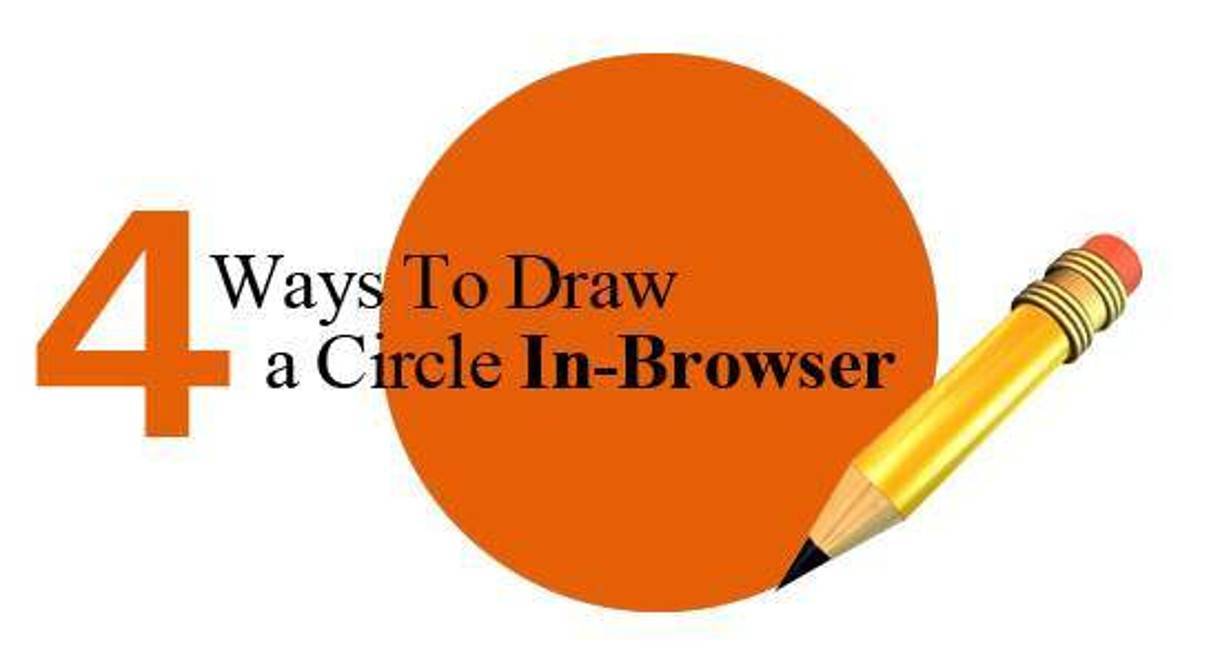
Four Ways to Draw a Circle in-Browser
At first glance, learning to program a circle in a browser is a task that to many would seem senseless. But believe it or not, creating a circle in a browser is a task that offers a lot of value to web designers of all skill levels.
Why take the time to render a circle with code, when we could simply use an image editing program like Photoshop to create the circle?
By rendering the circle in-browser, as opposed to embedding an image or coding it in flash, we ensure that it will be accessible from all devices (including Apple’s) and that there will be no loss in quality if the circle increases in size. Much like vector files used in Illustrator, with many of these methods, the circle can scale as the size of the browser increases. This becomes very useful in Mobile or Ipad application development, HTML5 games, and is a good base point for learning how to draw more complex objects.
Enough with the small talk, let’s get started examining the various ways of drawing a circle.
CSS
This method uses the border-radius property of CSS3, whose intended purpose is to round out the edges of a div or other element. The idea is to round out the edges to such an extent that the result looks something like a circle.
In order to get the shape to resemble a circle, this code required a tedious process of trial-and-error. To make it work, there were several CSS property values which had to be calculated and set.
/* css code */
div#css_example {
-moz-border-radius: 15px;
-webkit-border-radius: 15px;
border-radius: 15px;
border: solid 2px #000000;
background-color: #FF00FF;
width: 28px;
height: 28px;
margin-left: 25px;
margin-top: 15px;
}
Although some of these CSS properties could potentially be adjusted by the intervention of Javascript, the border-radius CSS property does not appear to have been intended for the purpose of drawing shapes. Complicating things further is IE’s lack of support for the border-radius CSS property for all releases until IE9. For these reasons, this method should only be regarded as a novelty or hack.
HTML5 Canvas
A more legitimate method to draw a circle is to use the HTML5 Canvas element. The first thing to be noted about this HTML5 method is that it does not have a built-in scene graph, which means that the image is not saved as a set of objects (i.e., a red circle here, a blue square there), but rather as a matrix of pixels.
In other words, if we were to draw a circle on one side of the screen and then move it to the other side, we cannot simply move the existing circle because the computer has no memory of the circle; there is only the memory of the bitmap that results from telling the computer to draw a circle. In this way, the method is comparable to drawing with MS Paint, except we’re using Javascript to draw things instead of the cursor.
/* javascript code */
var c = document.getElementById("html_canvas_example");
var ctx = c.getContext("2d");
ctx.fillStyle = "#FF00FF";
ctx.strokeStyle = "#000000";
ctx.lineWidth = 2;
ctx.beginPath();
ctx.arc(40,30,15,0,Math.PI*2,true);
ctx.closePath();
ctx.fill();
ctx.stroke();
For a more comprehensive list of browsers that support the canvas tag, check out this Wikipedia article.
SVG
Unlike the other three approaches discussed here, this method can be implemented without the intervention of a scripting language and entirely within the HTML document.
SVG’s, or Scalable Vector Graphics, are written using XML tags. Unlike the Canvas tag, SVG elements are remembered in the Document Object Model (DOM), which means that we can draw a circle on the screen and then refer to it again later by name.
One cool thing about SVG is that free tools are available to edit them, such as svg-edit. Using this browser-based application, a person can create a complex shape and then copy-and-paste the code that will recreate it in HTML.
<!-- HTML CODE -->
<svg xmlns="http://www.w3.org/2000/svg" version="1.1">
<circle cx="40" cy="30" r="15" fill="#FF00FF"
stroke="#000000" stroke-width="2" />
</svg>
Like the canvas tag and the CSS method discussed above, this approach is well-supported by all major browsers except for IE, which only began to support the most basics features of SVG in IE9. In addition, older versions of Android's default browser do not natively support SVG, although there are translation libraries available.
If interested in learning more about SVG, refer to w3schools.com.
Javascript libraries
The Raphael Javascript Library has quickly become one of our favorite tools for making online apps. There are many libraries like this one whose purpose is to improve the ease of creating graphics. This one does the job by interfacing with a combination of SVG and VM (Vector Markup). In this way, Raphael could be considered an extension of SVG.
/* javascript code */
// create the canvas
var paper = Raphael("raphael_example");
// draw a circle at screen position (40,30) with radius 15
var circle = paper.circle(40,30,15);// adjust the circle's attributes
circle.attr({
"fill" : "#FF00FF",
"stroke" : "#000000",
"stroke-width" : 2
});
Libraries like Raphael offer an elegant means of creating interactive graphics. However, they do so at a price. The source code which must be included weighs in at nearly 90 KB (minified). For users operating on older systems, the consequential loading time could be significant.
Because Raphael relies on SVG, it is bound to the limitations imposed by SVG—namely, a lack of all IE support except for IE9.
The Take-Away
If you’re creating complex images which require pixel-level manipulations, HTML Canvas might be for you. If, however, you’re making something that requires a degree of interactivity with the user, you may be better off using SVG (or one of its associated libraries, like Raphael). But it is wise to remember IE’s lack of SVG support.